- print#include <stdio.h>int main() {printf("Hello World!");return 0;}
- NewLine#include <stdio.h>int main() {printf("Hello World! \n");return 0;}
- Input#include <stdio.h>int main() {int Num;printf("Type a number: \n");scanf("%d", &Num);printf("number is: %d", Num);return 0;}
- Constantsconst int Num = 15;Num will always be 15
- sizechar alphabet[] = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";printf("%d", sizeof(alphabet));
Outputs 27sizeof will always return the memory size (in bytes) includes the \0 - Memory Addressint myAge = 23;printf("%p", &myAge);To access it, use the reference operator (&)
Outputs Memory Address like (0x7ffe5367e044)
- intstores integers (whole numbers), without decimals, such as 123 or -123int myNum = 1;
- floatstores floating point numbers, with decimals, such as 19.99 or -19.99float myFloatNum = 0.99;
- charstores single characters, such as 'a' or 'B'. Char values are surrounded by single quotesmyLetter = 'A';
- Type > %d or %iintint myNum = 1;
- Type > %ffloatfloat myFloatNum = 0.99;
- Type > %lfdoubledouble myDoubleNum = 19.99;
- Type > %ccharmyLetter = 'A';
- Type > %sstrings (text)char greetings[] = "Hello World!";
- BooleanA boolean data type value is returned, true = 1 and false = 0.bool isCodingFun = true
- Type Conversionint num1 = 9;int num2 = 2;float sum = (float) num1 / num2;printf("%f", sum);=>2.500000Operators
- Arithmetic Operators
Operators Name Example + Addition x + y - Subtraction x - y * Multiplication x * y / Division x / y % Modulus x % y ++ Increment x ++ y -- Decrement x -- y - Assignment Operators
Operators Example Same As = x = 10 y = 5 += x += 5 x = x + 10 -= x -= 5 x = x - 10 *= x *= 5 x = x * 10 /= x /= 5 x = x / 10 %= x %= 5 x = x % 10 ^= x ^= 5 x = x ^ 10 - Comparison Operators
Operators Name Example == Equal to x == y != Not Equal x != y > Greater than x > y < Less than x < y >= Greater than or equal to x >= y <= Less than or equal to x <= y - Logical Operators
Operators Name Example && Logical and x < 5 && x < 10 || Logical or x < 5 || x < 4 ! Logical Not !(x < 5 && x < 10)
If ... Else- ifUse if to specify a block of code to be executed, if a specified condition is trueif (condition) {}
- elseUse else to specify a block of code to be executed, if the same condition is falseif (condition) {} else { }
- else ifUse else if to specify a new condition to test, if the first condition is falseif (condition) {} else if (condition2) {} else {}
- Short Handint time = 20;if (time < 18) {printf("Good day.");} else {printf("Good evening.");(time < 18) ? printf("Good day.") : printf("Good evening.");
Loop- For Loopint i;for ( i = 0; i < 5; i++) {printf("%d\n", i);}
- Do While Loopint i = 0;do {printf("%d\n", i);i++;}while (i < 5);
- Nested Loopint i, j;for (i = 1; i <= 2; ++i) {printf("Outer: %d\n", i);for (j = 1; j <= 3; ++j) {printf(" Inner: %d\n", j);}}
Switch- Syntaxint day = 2;switch(day) {case 1:break;case 2:break;default:}
- Breakint i;for (i = 0; i < 10; i++) {if (i == 4) {break;}printf("%d\n", i);}
for loop break and jumps out when i is equal to 4: - Continueint i;for (i = 0; i < 10; i++) {if (i == 4) {Continue;}printf("%d\n", i);}
This example skips the value of 4:
Arrays- Syntaxint myNum[] = {10, 20, 30};;
Arrays are used to store multiple values or Elements in a single variable. - Access the Elementsint myNumbers[] = {25, 50, 75, 100};printf("%d", myNumbers[1]);
Outputs 50 - Change an Array Elementint myNumbers[] = {25, 50, 75, 100};myNumbers[0] = 33;printf("%d", myNumbers[0]);
Outputs 33 - Loop Through an Arrayint myNumbers[] = {25, 50, 75, 100};int i;for (int i = 0; i < 4; i++) {printf("%d\n", myNumbers[i]);}
- 2-Dimensional Arraysint matrix[2][3] = {{1, 4, 2} ,{3, 6, 8}};
Strings- Syntaxchar greetings[] = "Hello World!";char greetings[] = {'H', 'e', 'l', 'l', 'o','\0'};printf("%s", greetings);
must use the char type and create an array of characters to make a string in C. - Change & Access Elementschar greetings[] = "Hello World!";greetings[0] = 'J';printf("%c", greetings[0]);
Outputs J instead of H - Special Characters
character Result Description \' ' Single quote \" " Double quote \\ \ Backslash \n New Line \t Tab \0 Null - String Lengthchar alphabet[] = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";printf("%d", strlen(alphabet));
Outputs 26 - Concatenatechar str1[20] = "Hello ";char str2[] = "World!";strcat(str1, str2);printf("%s", str1);
Outputs 26 - Other FunctionsOther Functions like Concatenate areCopy Stringsstrcpy(str1, str2);Compare Stringsstrcmp(str1, str2);
Pointers- Syntax* Along with &
int myAge = 23;int* ptr = &myAge;
A pointer is a variable that stores the memory address of another variable as its value. - DereferenceAs syntax
printf("%p\n", ptr);Reference: Output memory address (0x7ffe5367e044)
printf("%d\n", *ptr);Dereference: Output Value (23) - Arraysint Numbers[4] = {25, 50, 75, 100};int i;for (i = 0; i < 4; i++) {printf("%p\n", &Numbers[i]);}
Outputs memory address of each - Related to Arraysint Numbers[4] = {25, 50, 75, 100};printf("%d\n", *(Numbers + 1));
Outputs 50 - loop throughint Numbers[4] = {25, 50, 75, 100};int *ptr = myNumbers;int i;for (i = 0; i < 4; i++) {printf("%d\n", *(ptr + i));
Functions- Syntaxvoid myFunction() { // Createprintf("I just got executed!");}int main() {myFunction(); // callreturn 0;}
- Parameters and Argumentsvoid Greet(char name[], char sname[]) {printf("Hello %s %s\n", name, sname);}int main() {Greet("Aditya","Roy");return 0; }name is a parameter, Aditya & Roy is a arguments.
- Pass Arraysvoid myFunction(int myNumbers[5]) {for (int i = 0; i < 5; i++) {printf("%d\n", myNumbers[i]);} }int main() {int myNumbers[5] = {10, 20, 30, 40, 50};myFunction(myNumbers);return 0; }
- Return Valuesint myFunction(int x) {return 5 + x;}int main() {printf("Result is: %d", myFunction(3));return 0;}
- Declaration and Definitionint myFunction(int, int); // declarationint main() {int result = myFunction(5, 3);printf("Result is = %d", result);return 0; }int myFunction(int x, int y) { // definitionreturn x + y;}
- Math Functions#includeprintf("%f", sqrt(16));
Function Description sqrt(x) square root of x pow(x,y) Xy abs(x) absolute value of x ceil(x) nearest up int floor(x) nearest down int cbrt(x) cube root of x
Recursion- SyntaxRecursion is the technique of making a function call itself. This technique provides a way to break complicated problems down into simple problems which are easier to solve.
- Exampleint sum(int);int main() {int result = sum(5);printf("Result is = %d", result);return 0;}int sum(int x) {if (k > 0) {return k + sum(k - 1);} else {return 0;}}
Files- File HandlingIn C, you can create, open, read, and write to files by declaring a pointer of type FILE, and use the fopen() function:FILE *fptrfptr = fopen(filename, mode);
filename mode like filename.txtlike filename.htmllike filename.cppw - Writes to a filea - Appends new data to a filer - Reads from a file - Create & close a FileTo create a file, you can use the w mode inside the fopen()fopen() function.However, if the file does not exist, it will create one for you.
FILE *fptr;fptr = fopen("filename.txt", "w");fclose(fptr);fclose() function will save & close the file. - Write To FilesTo insert content to it, you can use the fprint() functionFILE *fptr;fptr = fopen("C:\Aditya\filename.txt", "w");fprintf(fptr, "Hi Aditya");fclose(fptr);
Note: If you write to a file that already exists, the old content is deleted - Append Content To FilesIf you want to add content to a file without deleting the old content, you can use the a mode.FILE *fptr;fptr = fopen("C:\Aditya\filename.txt", "a");fprintf(fptr, "\nGood Moring");fclose(fptr);
- Read FilesFILE *fptr;fptr = fopen("C:\Aditya\filename.txt", "a");char myString[100];fgets(myString, 100, fptr);printf("%s", myString);fclose(fptr);we can use the fgets() function.Its takes three parameters
- Good ThingsIf you try to open a file for reading that does not exist, the fopen() function will return NULL. So we can use an if statement to test.if ( fptr == NULL ) {printf ("Not able to open the file.");}
C is a general-purpose programming language created by Dennis Ritchie at the Bell Laboratories in 1972. But i started learning in 2017-2018. It is a very popular language, despite being old.
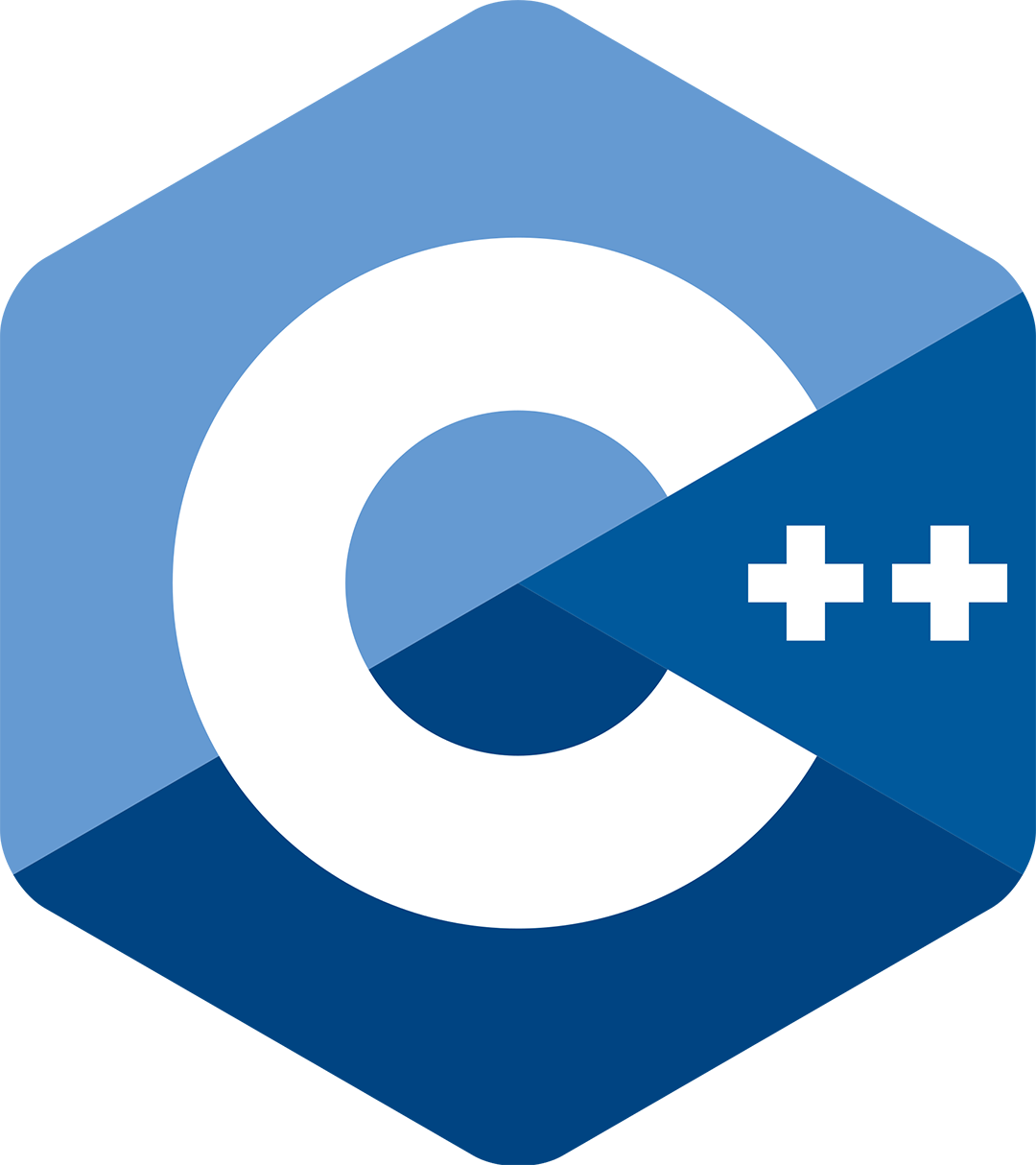
C ++
- print#include <iostream>using namespace std;int main() {cout << "Hello World!";return 0;}
- NewLine#include <iostream>using namespace std;int main() {cout << "Hello World! \n";cout << "Hello World!" << endl;return 0;}
- Input#include <iostream>using namespace std;int main() {int x;cout << "Type a number: \n";cin >> x;cout << "number is:", x);return 0;}
- Constantsconst int Num = 15;Num will always be 15
- Lengthstring txt = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";len = txt.length();len2 = txt.size();cout << len << len2
In both case Output will be same: 26
- intstores integers (whole numbers), without decimals, such as 123 or -123int myNum = 1;
- floatstores floating point numbers, with decimals, such as 19.99 or -19.99float myFloatNum = 0.99;
- charstores single characters, such as 'a' or 'B'. Char values are surrounded by single quotesmyLetter = 'A';
- stringstores text, such as "Hello World". String values are surrounded by double quotesstring myText = "Aditya";
- boolstores values with two states: true or falsebool myBoolean = true;
- intint myNum = 1;
- floatfloat myFloatNum = 0.99;
- doubledouble myDoubleNum = 19.99;
- charmyLetter = 'A';
- stringThe string type is used to store a sequence of characters (text). This is not a built-in type, but it behaves like one in its most basic usage. String values must be surrounded by double quotes:string greeting = "Hello";
- BooleanA boolean data type is declared with the bool keyword and can only take the values true or false. When the value is returned, true = 1 and false = 0.bool isCodingFun = true
- Arithmetic Operators
Operators Name Example + Addition x + y - Subtraction x - y * Multiplication x * y / Division x / y % Modulus x % y ++ Increment x ++ y -- Decrement x -- y - Assignment Operators
Operators Example Same As = x = 10 y = 5 += x += 5 x = x + 10 -= x -= 5 x = x - 10 *= x *= 5 x = x * 10 /= x /= 5 x = x / 10 %= x %= 5 x = x % 10 ^= x ^= 5 x = x ^ 10 - Comparison Operators
Operators Name Example == Equal to x == y != Not Equal x != y > Greater than x > y < Less than x < y >= Greater than or equal to x >= y <= Less than or equal to x <= y - Logical Operators
Operators Name Example && Logical and x < 5 && x < 10 || Logical or x < 5 || x < 4 ! Logical Not !(x < 5 && x < 10) - Bitwise operators
Operators inpu1 inpu2 Output & 0101 0110 0100 | 0101 0110 0111 ^ 0101 0110 0011 ~ 0101 1010
- ifUse if to specify a block of code to be executed, if a specified condition is trueif (condition) {}
- elseUse else to specify a block of code to be executed, if the same condition is falseif (condition) {} else { }
- else ifUse else if to specify a new condition to test, if the first condition is falseif (condition) {} else if (condition2) {} else {}
- Short Handint time = 20;if (time < 18) {cout << "Good day.";} else {cout << "Good evening."; }string result = (time < 18) ? "Good day." : "Good evening.";cout << result;
- For Loopint i;for ( i = 0; i < 5; i++) {cout << i << "\n";}
- Do While Loopint i = 0;do {cout << i << "\n";i++;}while (i < 5);
- Nested Loopint i, j;for (i = 1; i <= 2; ++i) {cout << "Outer: " << i << "\n";for (j = 1; j <= 3; ++j) {cout << " Inner: " << j << "\n";}}
- Foreach Loopint myNumbers[5] = {10, 20, 30, 40, 50};for (int i : myNumbers) {cout << i << "\n";}
- Syntaxint day = 1;switch(day) {case 1:break;case 2:break;default:}
- Breakfor (int i = 0; i < 10; i++) {if (i == 4) {break;}cout << i << "\n";}
for loop break and jumps out when i is equal to 4: - Continuefor (int i = 0; i < 10; i++) {if (i == 4) {Continue;}cout << i << "\n";}
This example skips the value of 4:
- Syntaxstring char[4];string char[4] = {"A", "B", "C", "D"};int myNum[3] = {10, 20, 30};;
Arrays are used to store multiple values or Elements in a single variable. - Access the Elementsstring char[4] = {"A", "B", "C", "D"};cout << char[0];
Outputs A - Change an Array Elementstring char[4] = {"A", "B", "C", "D"};char[0] = "E";cout << char[0];
Outputs E - Loop Through an Arraystring char[4] = {"A", "B", "C", "D"};for (int i = 0; i < 5; i++) {cout << cars[i] << "\n";}
- 2-Dimensional Arraysstring letters[2][4] = {{ "A", "B", "C", "D" },{ "E", "F", "G", "H" }};
- 3-Dimensional Arraysstring letters[2][2][2] = {;{ { "A", "B" } ,{ "C", "D" } } ,{ { "E", "F" } ,{ "G", "H" } },};
- Syntaxstring char[4];string char[4] = {"A", "B", "C", "D"};
String it self an array,there is nothing change from c++ array.c++ String upgrade of c .so most Function are same - Input Stringsstring firstName;cout << "Type your full name: ";getline (cin, fullName);cout << "Your name is: " << fullName;
If we use cin but it takes the first parameter - Concatenationstring fName = "Aditya";string lName = "Roy";string fullName = fName+" "+lName;string fullName = fName.append(lName);cout << fullName;
outputs both: Aditya Roy - Other FunctionsCompare Stringsn1.compare(n2)to clear everythingn1.clear()to check n1 is empty or notn1.empty()to erase some word from n1n1.erase(3,3) //start,uptoto Findn1.find("something")
- Other Functions 2to insertn1.insert(2,"lol")to substractionn1.substr(6,4)//start,uptostrings to integersx =stoi(n1)integers to stringsx =to_string(n1)sorting stringssort(n1.begin(),n1.end())
- Other Functions 3Upper casen[i]-=32 //loop 1 by 1transform(n1.begin(),n1.end(), n1.begin(),::toupper)lower casen[i]+=32 //loop through 1 by 1transform(n1.begin(),n1.end(), n1.begin(),::tolower)
- Syntax* Along with &
string food = "Pizza";string* ptr = &food;
A pointer is a variable that stores the memory address of another variable as its value. - DereferenceAs syntax
cout << ptr << "\n";Reference: Output memory address (0x6dfed4)
cout << *ptr << "\n";Dereference: Output Value (Pizza) - Modifystring food = "Pizza";string* ptr = &food;cout << *ptr << "\n";
Outputs Pizza
*ptr = "Hamburger";cout << *ptr << "\n";
Outputs Hamburger - ways to declare pointerstring* mystring; ( Preferred )string *mystring;string * mystring;
- Syntaxvoid myFunction() { // Createcout << "I just got executed!";}int main() {myFunction(); // callreturn 0;}
- Parameters and Argumentsvoid myFunction(string fname. string sname) {cout << fname << sname << " Bro\n";}int main() {Greet("Aditya","Roy");return 0; }fname is a parameter, Aditya & Roy is a arguments.
- Pass Arraysvoid myFunction(int myNumbers[5]) {for (int i = 0; i < 5; i++) {cout << myNumbers[i] << "\n";} }int main() {int myNumbers[5] = {10, 20, 30, 40, 50};myFunction(myNumbers);return 0; }
- Return Valuesint myFunction(int x) {return 5 + x;}int main() {cout << myFunction(3);return 0;}
- Declaration and Definitionint myFunction(int, int); // declarationint main() {int result = myFunction(5, 3);cout <<"Result is :", result;return 0; }int myFunction(int x, int y) { // definitionreturn x + y;}
- Default Parametervoid myFunction(string country = "Norway"){cout << country << "\n"; }int main() {myFunction("India");myFunction();return 0; }Here = "Norway" default value of country
- SyntaxRecursion is the technique of making a function call itself. This technique provides a way to break complicated problems down into simple problems which are easier to solve. Recursion may be a bit difficult to understand. The best way to figure out how it works is to experiment with it.
- Exampleint sum(int x) {if (k > 0) {return k + sum(k - 1);} else {return 0;}}int main() {int result = sum(10);cout << result;return 0;}
- File HandlingIn C++,The fstream library allows us to work with files. To use the fstream library, include both the standard iostream iostream AND the fstream header file:
Class Description ofstream Creates and writes ifstream Reads from files fstream Both - Create & close a FileTo create a file, use either the ofstream fstream class, and specify the name of the file.
ofstream MyFile("filename.txt");MyFile.close();.close() function will save & close the file. - Write To FilesTo write to the file, use the insertion operator <<.
ofstream MyFile("filename.txt");MyFile << "Files can be tricky";MyFile.close(); - Read Filesstring myText;ifstream MyReadFile("filename.txt");while (getline (MyReadFile, myText)) {cout << myText;}MyReadFile.close();we can use the getline() function.
C++ is a popular programming language created by Bjarne Stroustrup in 1985. But i started learning in 2019-2020 . C++ is a cross-platform language that can be used to create high-performance applications.
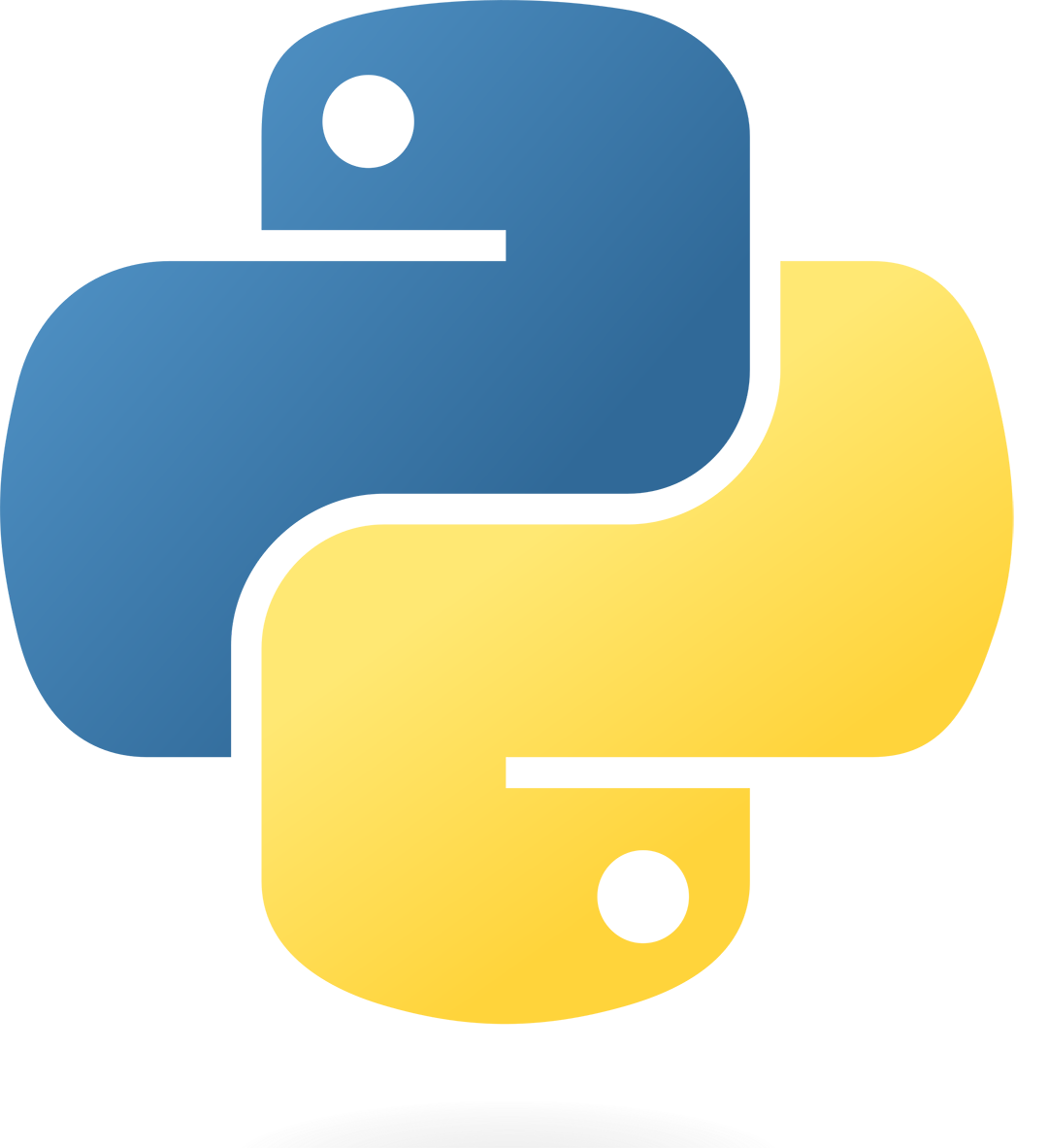
Py thon
- printprint("Hello, World!")
- InputPython 3.6username = input("Enter name:")
Python 2.7username = raw_input("Enter name:") - NewLineprint("Hello, World!" \n)print(end="Hello, World!")Or we can write like this :mystring = """Hello1st2nd3rd. """
- Lengthword= "ABCDEFGHIJKLMNOPQRSTUVWXYZ";x = len(word)print(x)
Output: 26 - dict to dataframeimport pandas as pdany nested Dictionariesdf=pd.DataFrame(myfamily)print(df)
- intstores integers (whole numbers), without decimals, such as 123 or -123x = 1
- floatstores floating point numbers, with decimals, such as 19.99 or -19.99x = 2x = 2.00
- stringstores text, such as "Aditya". String values are surrounded by double quotesx = "Aditya"x = 'Aditya'
- Variable NamesVariable names are case-sensitive.A variable name must start with a letter or the underscore character like:-My_2nd_name = "Atomic"
- Assign Multiple ValuesMany Values to Multiple Variablesx, y, z = "Orange", "Banana", "Cherry"One Value to Multiple Variablesx = y = z = "Orange"Unpack a Collectionfruits = ["apple", "banana", "cherry"]x, y, z = fruits
- Global Variablesx = "awesome" #Globadef myfunc():global x #to change globalx = "fantastic"myfunc()print("Python is " + x)
- Text Type:1. x = str("Hello World")x = "Hello World"
- Mapping Type:1. x= dict(name="John", age=36)x= {"name" : "John", "age" : 36}
- Boolean Type:1. x= bool(5). x= True
- Numeric Types:1. x = int(20)x = (202. x = float(20.5)x = 20.53. x = complex(6k)x = 6k
- Sequence Types:1. x= list(("apple", "banana", "cherry"))x= ["apple", "banana", "cherry"]2. x= tuple(("apple", "banana", "cherry"))x= ("apple", "banana", "cherry")3. x= range(6)
- Binary Types:1. x= bytes(5)x= b"Hello"2. x= bytearray(5)3. x= memoryview(bytes(5))
- Set Types:1. x= set(("apple", "banana", "cherry"))x= {"apple", "banana", "cherry"}2. x= frozenset(("apple", "banana", "cherry"))x= ({"apple", "banana", "cherry"})
- None Type:1. x= None
- Arithmetic Operators
Operators Name Example + Addition x + y - Subtraction x - y * Multiplication x * y / Division x / y % Modulus x % y ** Exponentiation x ** y // Floor division x // y - Assignment Operators
Operators Example Same As = x = 10 y = 5 += x += 5 x = x + 10 -= x -= 5 x = x - 10 *= x *= 5 x = x * 10 /= x /= 5 x = x / 10 %= x %= 5 x = x % 10 ^= x ^= 5 x = x ^ 10 - Comparison Operators
Operators Name Example == Equal to x == y != Not Equal x != y > Greater than x > y < Less than x < y >= Greater than or equal to x >= y <= Less than or equal to x <= y - Logical Operators
Operators Name Example and Logical and x < 5 and x < 10 or Logical or x < 5 or x < 4 not Logical Not not(x < 5 && x < 10) - Identity & Membership
Operators Description Example is Returns True if x = y x is y is not Returns True if x not= y x is not y in Returns True if x presenr in y x in y not in Returns True if x not presenr in y x not in y - Bitwise operators
Ope inpu1 inpu2 exp Output & 0101 0110 x & y 0100 | 0101 0110 x | y 0111 ^ 0101 0110 x ^ y 0011 ~ 0101 ~ x 1010 << 0011 x << 2 1100 >> 1100 x >> 2 0011
- ifUse if to specify a block of code to be executed, if a specified condition is trueif condition:
Short Handif a > b: print("a is greater than b") - elseUse else to specify a block of code to be executed, if the same condition is falseif condition:else:
- elifUse else if to specify a new condition to test, if the first condition is falseif condition:elif condition2:} else:
Short Handif a > b: print("a is greater than b")
print("A") if a > b else print("=") if a == b else print("B")
- For Loopfruits = ["apple", "banana", "cherry"]for x in fruits:print(x)
- For Loop a stringfor x in "banana":print(x)
- For Loop a rangefor x in range(2, 30, 3):print(x)
- While Loopi = 0;while i < 6:print(i)i += 1
- Nested Loopadj = ["red", "big", "tasty"]fruits = ["apple", "banana", "cherry"]for x in adj:for y in fruits:print(x, y)
- Else in For Loopfor x in range(6):print(x)else:print("Finally finished!")
- Basicthisdict = {"brand": "Ford","model": "Mustang","year": 1964 }print(thisdict)
- Accessing Itemsthisdict = {"brand": "Ford","model": "Mustang","year": 1964 }print(thisdict["brand"])
- Change or addthisdict = {"brand": "Ford","model": "Mustang","year": 1964 }thisdict["year"] = 2020thisdict.update({"year": 2020})
- Removing Itemsthisdict = {"brand": "Ford","model": "Mustang","year": 1964 }thisdict .pop("model")del thisdict["model"]
- Copy Dictionariesthisdict = {"brand": "Ford","model": "Mustang","year": 1964 }mydict = thisdict .copy()mydict = dict(thisdict)print(mydict)
- Nested Dictionariesmyfamily = {"child1" : {"name" : "Aditya","year" : 1998 },"child2" : {"name" : "Abhijit","year" : 2003 },}print(myfamily["child2"]["name"])
- Syntaxlambda arguments : expression
x = lambda a : a + 10print(x(5))
Add 10 to argument a, and return the result: - Multiply argumentx = lambda a, b : a * bprint(x(5, 6))
Multiply argument a with argument b and return the result: - Summarize argumentx = lambda a, b, c : a + b + cprint(x(5, 6, 2))
Summarize argument a, b, c and return the result: - Use Lambdadef myfunc(n):return lambda a : a * n
mydoubler = myfunc(2)mytripler = myfunc(3)
print(mydoubler(11))print(mytripler(11))
- Syntaxcars = ["Ford", "Volvo", "BMW"]
Arrays are used to store multiple values or Elements in a single variable. - Access the Elementscars = ["Ford", "Volvo", "BMW"]x = cars[0]print(x)
Outputs: Ford - Change an Array Elementcars = ["Ford", "Volvo", "BMW"]cars[0] = "Toyota"x = cars[0]print(x)
Outputs: Toyota - Loop Through an Arraycars = ["Ford", "Volvo", "BMW"]for x in cars:print(x)
- Array Methodscars = ["Ford", "Volvo", "BMW"]cars.append("Honda")print(cars)
output : ['Ford', 'Volvo', 'BMW', 'Honda'] - All Array Methods
Method Method append() pop() clear() copy() count() extend() index() insert() remove() reverse() sort()
- Syntaxa="Hello"print("Hello")print('Hello')
single or double quotation marks.both sameYou can display a string literal with the print() function: - Slicing Stringsa = "Hello, World!"print(a[2:5])
outputs: lloa[ start : end ] - ConcatenationfName = "Aditya";lName = "Roy";fullName = fName+" "+lNameprint(fullName)
output: Aditya Roy - String Formatage = 24year = 2023txt = "My name is Aditya, and I am {} at {}"print(txt.format(age,year))
output: My name is Aditya, and I am 24 at 2023
- Syntaxdef my_function(): // Createprint("Hello from a function")
my_function() // call - Argumentsdef my_function(fname, lname):print(fname + " " + lname)
my_function("Aditya","Roy") - Keyword Argumentsdef my_function(child3, child2, child1):print("The youngest child is " + child3)my_function(child1 = "Emil", child2 = "Tobias", child3 = "Linus")
- Return Valuesdef my_function(x):return 5 * x
print(my_function(3)) - Arbitrary Keyworddef my_function(**kid):print("His last name is " + kid["lname"])
my_function(fname = "Tobias", lname = "Refsnes") - Default Parameterdef my_function(country = "Norway"):print("I am from " + country)my_function("India")
Here = "Norway" default value of country
- SyntaxRecursion is the technique of making a function call itself. This technique provides a way to break complicated problems down into simple problems which are easier to solve. Recursion may be a bit difficult to understand. The best way to figure out how it works is to experiment with it.
- Exampledef recursion(k):if(k > 0):result = k + recursion(k - 1)print(result)else:result = 0return result
print("\n\nRecursion Example Results")recursion(6)
- File HandlingThe key function for working with files in Python is the open() function.x = open("filename", "mode");
filename mode like filename.txtlike filename.htmllike filename.cppw - Writes to a filea - Appends new data to a filer - Reads from a filex - Create a filet - Text modeb - Binary mode - Create & close a FileThe open() function returns a file object, which has a read() method for reading the content of the file:
f = open("demofile.txt", "rt")f.close()
f.close() function will save & close the file. - Write To Filesf = open("demofile2.txt", "a")f.write("Now the file has more content!")f.close()
Note: a method use for Append . if we use w method that will overwrite the entire file. - Read Filesf = open("D:\\myfiles\welcome.txt", "r")print(f.read())
we can also use the f.read(5) readline() function. and also a Loop for x in f: print(x) - Delete a FileTo delete a file or folder, you must import the OS module, and run its os.remove() function.
import osos.remove("demofile.txt")
os.rmdir("myfolder") - Check to deleteimport osif os.path.exists("demofile.txt"):os.remove("demofile.txt")else:print("The file does not exist")
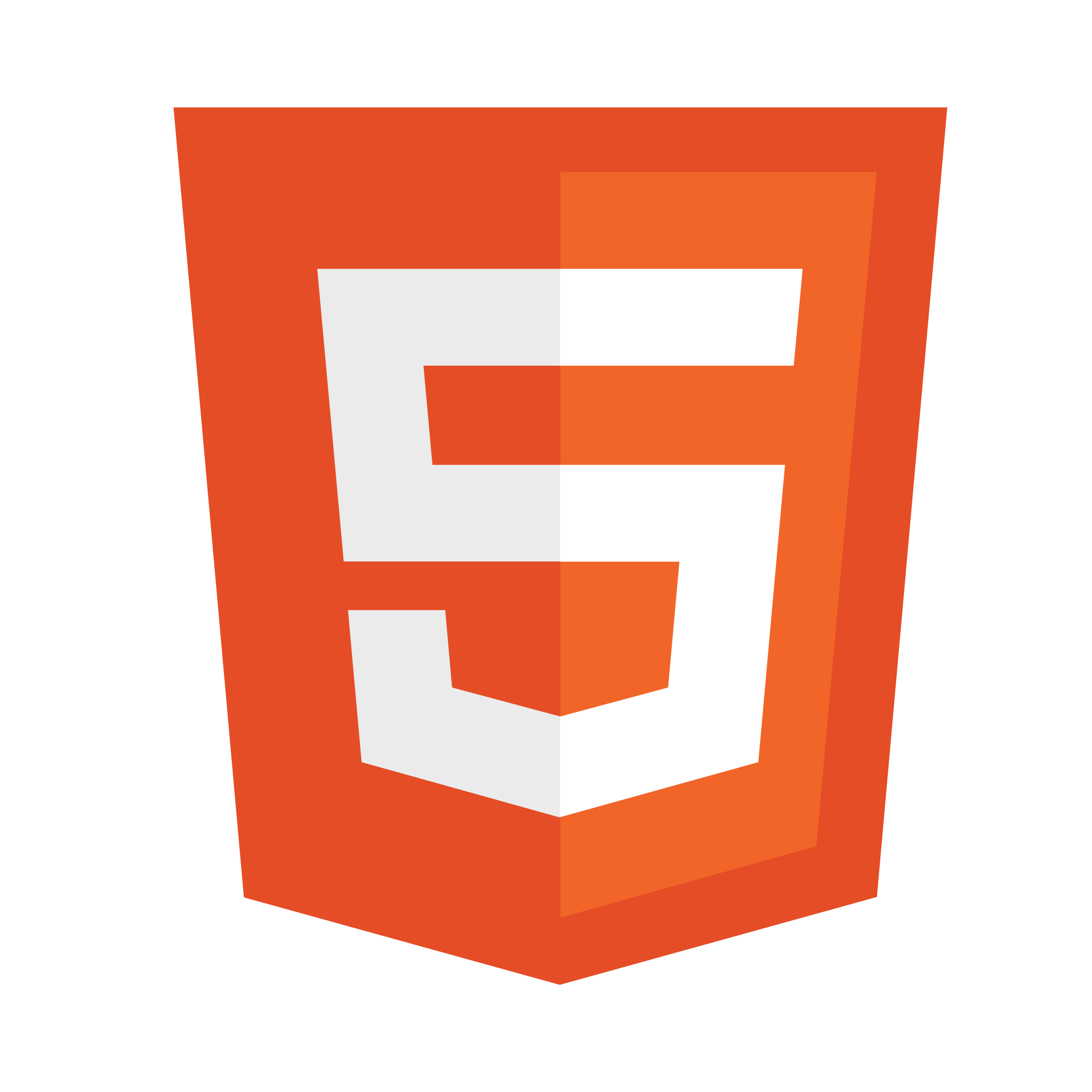
HT ML
- MainThe <!DOCTYPE> declaration represents the document type, and helps browsers to display web pages correctly.The <!DOCTYPE> declaration for HTML5 is:<!DOCTYPE html>The <br> element called empty elements
- HTML tags1. HTML headings: <h1> to <h6> tags.2. HTML paragraphs: <p>tags.3. HTML links : <a> tags.destination is specified in the href attribute.4. HTML images: <img> tags. The source file (src), alternative text (alt) are provided as attributes.
- HTML Attributes1. We already know href ,src & alt Attributes2. The style attribute is used to add styles to an element, such as color, font, size, and more.3. The title attribute defines some extra information about an element.
- Text Formatting
Tag Description <b> Bold text <strong> Important text <i> Italic text <em> Emphasized text <mark> Marked text <small> Smaller text <del> Deleted text <ins> Inserted text <sub> Subscript text <sup> Superscript text - target AttributeThe target attribute specifies where to open the linked document.1. _self Default. Opens the document in the same window/tab as it was clicked2. _blank Opens the document in a new window or tab3. _parent Opens the document in the parent frame4. _top Opens the document in the full body of the window
iconlink rel="icon" type="image/x-icon" href=""> - scriptThe HTML <script> tag is used to define a client-side script (JavaScript).The <script> element either contains script statements, or it points to an external script file through the src attribute.
- Syntax<table><tr><th><th></tr><tr><td><td></tr></table>
- explanation1. Table start with<Table> and end with </Table> tag2. Each table row starts with a <tr> and ends with a </tr> tag3. Sometimes you want your cells to be table header cells. In those cases use the <th> and ends with a </th> tag4. <td> stands for table data.Everything between <td> and </td> are the content of the table cell.
- Columns span<table><tr><th colspan="2"><th></tr><tr><td><td><td></tr></table>
- Rows span<table><tr><th rowspan="2"><th></tr><tr><td><td><td></tr></table>
- Table Caption<table><caption> caption </caption><tr><th><th></tr><tr><td><td><td></tr></table>
- Colgroup<table><colgroup><col span="2"></colgroup><tr><th><th></tr><tr><td><td><td></tr></table>
- Ordered ListAn ordered list starts with the ol tag. Each list item starts with the li tag.<ol><li><li></ol>
- UnOrdered ListAn unordered list starts with the ul tag. Each list item starts with the li tag.<ul><li><li></ul>
- List itemEach list item starts with the li tag.
- Description ListAn Description list starts with the dl tag.<dl><dt><dd></dl>
- Description term (name)Defines a term in a description list starts with the dt tag.
- Description describesDescribes the term in a description list starts with the dd tag.
- Block-levelA block-level element always starts on a new line, and the browsers automatically add some space (a margin) before and after the element.A block-level element always takes up the full width available (stretches out to the left and right as far as it can).Two commonly used block elements are: <p> and ,<div>.
- Inline ElementsAn inline element does not start on a new line.An inline element only takes up as much width as necessary.some commonly used inline elements are: <span> ,<input> ,<button>.
- classThe HTML class attribute is used to specify a class for an HTML element.The HTML class attribute is used to specify a class for an HTML element.The class attribute is often used to point to a class name in a style sheet. It can also be used by a JavaScript to access and manipulate elements with the specific class name.To create a class; write a period (.) character, followed by a class name. Then, define the CSS properties within curly braces {}:
Syntax< div class="city" >< /div >
css.city {} - idThe HTML id attribute is used to specify a unique id for an HTML element.You cannot have more than one element with the same id in an HTML document.The id attribute is used to point to a specific style declaration in a style sheet. It is also used by JavaScript to access and manipulate the element with the specific id.The syntax for id is: write a hash character (#), followed by an id name. Then, define the CSS properties within curly braces {}.
Syntax< h1 id="myHeader" > My Header< /h1 >
css#myHeader{} - iframeThe HTML iframe attribute is used to display a web page within a web page.
Syntax< iframe src="url" title="description" >< /iframe >
cssiframe {}
- Layout Elements
Elements Elements < header > < nav > < section > < article > < aside > < footer > < details > < summary > - Layout TechniquesCSS float propertyCSS flexboxCSS gridCSS framework
- CSS FloatIt is common to do entire web layouts using the CSS float property. Float is easy to learn - you just need to remember how the float and clear properties work. Disadvantages: Floating elements are tied to the document flow, which may harm the flexibility.
- CSS FlexboxUse of flexbox ensures that elements behave predictably when the page layout must accommodate different screen sizes and different display devices.
- CSS GridThe CSS Grid Layout Module offers a grid-based layout system, with rows and columns, making it easier to design web pages without having to use floats and positioning.
- CSS FrameworksIf you want to create your layout fast, you can use a CSS framework, like Bootstrap.
- kbdThe < kbd > element defines keyboard input
< p > Save the document by pressing < kbd > Ctrl + S < /kbd >< /p >
Save the document by pressing Ctrl + S
- sampThe < samp > element defines sample output from a computer program
< p>< samp >File not found.< br>Press F1 to continue< /samp >< /p>
File not found.
Press F1 to continue - codeThe < code > element defines a piece of computer code
< code >x = 5;y = 6;z = x + y;< /code >
x = 5; y = 6; z = x + y;
- preThe < pre > element defines preformatted text
< pre >< code >x = 5;y = 6;< /code >< /pre >
x = 5;y = 6;
- Syntax< form >
form elements
< /form > - Mian < input > Elementinput type="text"input type="radio"input type="checkbox"input type="submit"input type="button"
- Form Attributes1. The action attribute defines the action to be performed when the form is submitted.2. Target Attribute
_blank _self _parent _top framename - Elementsinputlabelselectoptiontextareabuttonfieldsetlegenddatalist
- Input Types< input type="color" >< input type="date" >< input type="email" >< input type="file" >< input type="image" >< input type="number" >< input type="url" >< input type="password" >< input type="time" >< nput type="range" >
- placeholder AttributeThe input placeholder attribute specifies a short hint that describes the expected value of an input field
- circlecircle cx="50" cy="50" r="40" stroke="green" stroke-width="4" fill="yellow"1. The cx and cy attributes define the x and y coordinates of the center of the circle2. The r attribute defines the radius of the circle
- Rectanglerect x="50" y="20" rx="20" ry="20" width="150" height="150"1. The x and y attributes define the left and top position of the rectangle1. The rx and ry attributes rounds the corners of the rectangle
- ellipseellipse cx="200" cy="80" rx="100" ry="50"1. The cx and cy attributes define the x and y coordinates of the center of the ellipse1. The rx attributes defines the horizontal radius1. The ry attributes defines the vertical radius
- polygonpolygon points="100,10 40,198 190,78 10,78 160,198"The points attribute defines the x and y coordinates for each corner of the polygon
- lineline x1="0" y1="0" x2="200" y2="200"1. The x1 and y1 attribute defines the start of the line on the x-axis and Y-axis2. The x2 and y2 attribute defines the end of the line on the x-axis and Y-axis
- polylinepolyline points="0,40 40,40 40,80 80,80 80,120 120,120 120,160"The points attribute defines the list of points (pairs of x and y coordinates) required to draw the polyline
HTML is the standard markup language for Web pages. created by Tim Berners-Lee in 1993. But i start learning in 2023 .
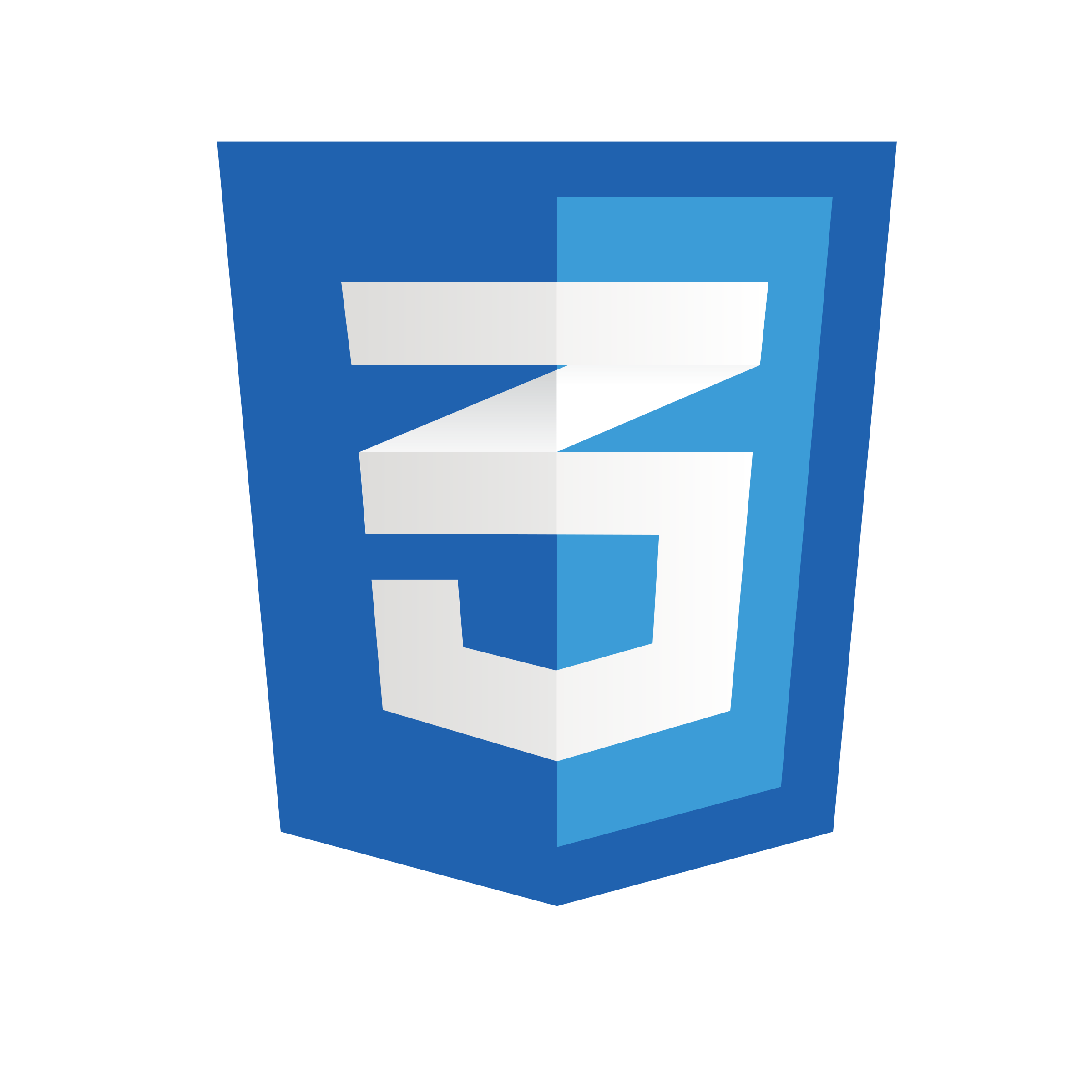
C ss
- css1. CSS stands for Cascading Style Sheets2. CSS describes how HTML elements are to be displayed on screen, paper, or in other media3. CSS saves a lot of work. It can control the layout of multiple web pages all at once4. External stylesheets are stored in CSS files
- SyntaxIn this example all <p> elements will be center-aligned, with a red text color:
p {color: red;text-align: center;}
- Simple selectorsselect elements based on name, id, class
In html In css < p > p { } id="para1" #para1 { } class="center" .center { } < p class="cen" > p.cen { } All html * { } h1 h1 { } - Combinator selectorsselect elements based on a specific relationship between them
Name css Descendant Selector div p { } Child Selector (>) div > p { } Adjacent Sibling Selector (+) div + p { } General Sibling Selector (~) div ~ p { } - Pseudo-class selectorsA pseudo-class is used to define a special state of an element.
Name In css Anchor a:link { } a:hover { } first-child p:first-child { } p i:first-child { } - Pseudo-elements selectorsA CSS pseudo-element is used to style specified parts of an element.
In html In css < p > p::first-line { } p.intro::first-letter { } < h1 > h1 : : before { } h1: : after { } (no need) : : marker { } (no need) : : selectio { } - Attribute SelectorsIt is possible to style HTML elements that have specific attributes or attribute values.
In html In css < a target=""> a [ target ] { } title = "flower" [ title ~ =" flower " ] { } class " top-text ". [class | " top " ] { } [ class ^ = "top" ] { } class = " mytest " [ class $ = " test " ] { } [ class * = " te " ] { }
- Color NamesIn CSS, a color can be specified by using a predefined color name:red
- Background ColorYou can set the background color for HTML elements:background-color: DodgerBlue;
- Border ColorYou can set the color of borders:border:2px solid Tomatoborder:2px solid Violet
- outline ColorYou can set the color of outline:
outline: 1px solid yellow;
outline: dotted red; - Color Valuesrgb(250,90,70,1)#b8f050yellow
- background-colorbackground-color: red;background-color: #ff0000;background-color: rgb(255,0,0);
- GradientsLinear Gradients (goes down/up/left/right/diagonally)Radial Gradients (defined by their center)Conic Gradients (rotated around a center point)
background-image: linear-gradient(red, yellow); - background-imagebackground-image: url("aditya.jpg");background-repeat: no-repeat;background-position: right top;
- background-attachmentbackground-image: url("aditya.jpg");background-repeat: no-repeat;background-attachment: fixed;
- elementbackground-size: cover;background-origin: border-box;background-clip: content-box;
- Multiple + Shorthandbackground: url(aditya.jpg) right bottom no-repeat, url(aditya2.jpg) left top repeat;
- ColorThe color property is used to set the color of the text. The color is specified by:a color name - like "red"a HEX value - like "#ff0000"an RGB value - like "rgb(255,0,0)"
- Alignmenttext-alignThe text-align property is used to set the horizontal alignment of a text.like center
text-align-lastThe text-align-last property specifies how to align the last line of a text. like right - DirectiondirectionThe direction and unicode-bidi properties can be used to change the text direction of an element.direction: rtl;unicode-bidi: bidi-override;
VerticalThe Vertical Alignment property sets the vertical alignment of an element. - Decorationtext-decoration-line : overline
text-decoration-color : red; (+ line)
text-decoration-style: wavy; (+ line)
text-decoration-thickness: auto; (+ line)Short hand :- text-decoration: underline aqua double 3px; - Spacingtext-indent: 50px;letter-spacing: 5px;line-height: 1.8;word-spacing: 10px;white-space: nowrap;
- Shadowtext-shadow: 1px 1px; (x,y)text-shadow: 1px 1px aqua;text-shadow: 1px 1px 5px aqua;(x,y,blur)text-shadow: 1px 1px 2px yellow, 0 0 25px greenyellow, 0 0 5px green;
- Fontsfont-family: "Times New Roman", Times, serif;font-style: italic;font-weight: bold;font-variant: small-caps;font-size: 15px;shorthand: font: italic small-caps bold 12px/15px Georgia, serif;
- Linksa:link - a normal, unvisited linka:visited - a link the user has visiteda:hover - a link when the user mouses over ita:active - a link the moment it is clicked
- Listslist-style-type: circle;list-style-image: url();list-style-position: inside;shorthand: list-style: square inside url();
- Tablesborder-collapse: collapse;border-bottom: 1px solid #ddd;tr:hover {background-color: coral;}
- displayDisplay: none;
visibility: hidden; - z-indexThe z-index property specifies the stack order of an element (which element should be placed in front of, or behind, the others).
If two positioned elements overlap each other without a z-index specified, the element defined last in the HTML code will be shown on top.
- static1. HTML elements are positioned static by default.2. An element with position: static; is not positioned in any special way; it is always positioned according to the normal flow of the page:position: static;border: 3px solid yellow;
- relativeAn element with position: relative; is positioned relative to its normal position.position: relative;top: 30px;border: 3px solid aqua;
- fixedAn element with position: fixed; is positioned relative to the viewport, which means it always stays in the same place even if the page is scrolled.position: fixed;top: 40vh;90hvright: 10vw;width: 300px;/150pxborder: 3px solid purple;
- absoluteAn element with position: absolute; is positioned relative to the nearest positioned ancestor (instead of positioned relative to the viewport, like fixed).position: absolute;top: 12vh;left: 1vw;width: 300px;border: 3px solid greenyellow;
- stickyAn element with position: sticky; is positioned based on the user's scroll position.
A sticky element toggles between relative and fixed, depending on the scroll position. It is positioned relative until a given offset position is met in the viewport - then it "sticks" in place (like position:fixed).
- floatnone - The element does not float (will be displayed just where it occurs in the text). This is defaultleft - The element floats to the left of its containerright - The element floats to the right of its containerinherit - The element does not float (will be displayed just where it occurs in the text). This is default
- clearnone - The element is not pushed below left or right floated elements. This is defaultleft - The element is pushed below left floated elementsright - The element is pushed below right floated elementsboth - The element is pushed below both left and right floated elementsinherit - The element inherits the clear value from its parent
- clearfix HackIf a floated element is taller than the containing element, it will "overflow" outside of its container. We can then add a clearfix hack to solve this problem:
.clearfix {overflow: auto; }
.clearfix::after {content: "";clear: both;display: table; }
- Absolute LengthsThe absolute length units are fixed and a length expressed in any of these will appear as exactly that size.
Unit Description cm centimeters mm millimeters in inches (1in = 96px = 2.54cm) px pixels (1px = 1/96th of 1in) pt points (1pt = 1/72 of 1in) pc picas (1pc = 12 pt) - Relative LengthsRelative length units specify a length relative to another length property. Relative length units scale better between different rendering mediums.
Unit Description em Relative to the font-size of the element (2em means 2 times the size of the current font) rem Relative to font-size of the root element ex Relative to the x-height of the current font (rarely used) ch Relative to width of the "0" (zero) px pixels (1px = 1/96th of 1in) pt points (1pt = 1/72 of 1in) pc picas (1pc = 12 pt)
- calc( )The calc() function performs a calculation to be used as the property value.
CSS Syntaxcalc(expression)
calc(100% - 100px); - max( )The max() function uses the largest value, from a comma-separated list of values, as the property value.
CSS Syntaxmax(value1, value2, ...)
max(50%, 300px); - min( )The min() function uses the smallest value, from a comma-separated list of values, as the property value.
CSS Syntaxmin(value1, value2, ...)
min(50%, 300px);
- Syntaxborder-radius: 25px;background: #73AD21;padding: 20px;the value applies to all four corners, which are rounded equally
- Four valuesfirst value applies to top-left corner, second value applies to top-right corner, third value applies to bottom-right corner, and fourth value applies to bottom-left cornerborder-radius: 15px 50px 30px 5px;background: aqua;padding: 20px;
- Three valuesfirst value applies to top-left corner, second value applies to top-right and bottom-left corner, third value applies to bottom-right cornerborder-radius: 15px 50px 30px;background: yellowgreen;padding: 20px;
- Two valuesfirst value applies to top-left and bottom-right corner, second value applies to top-right and bottom-left cornerborder-radius: 15px 50px;background: purple;padding: 20px;
- elliptical cornersborder-radius: 25px / 50px;background: red;padding: 20px;
- box-shadowbox-shadow: 10px 10px 5px 12px lightblue;x , y , colorx , y , blur , colorx , y , blur , spread , color
- Image Reflection-webkit-box-reflect: below;
-webkit-box-reflect: below 0px linear-gradient(to bottom, rgba(0,0,0,0.0), rgba(0,0,0,0.4)); - objectobject-fit1. fill2. contain3. cover4. scale-down
object-position: x y ; - Multiple Columnscolumn-count:3;column-gap:10px;column-rule:1px solid lightblue;column-span:allcolumn-width:100px
- VariablesThe var( ) function is used to insert the value of a CSS variable.;Syntax:- var(--name, value)Global:- :root { var ( ) }Local:- class/id { var ( ) }
- Media Queries@media not|only mediatype and (expressions) { CSS-Code; }all - Used for all media type devicesprint - Used for printersscreen - Used for computer screens, tablets, smart-phones etc.speech - Used for screenreaders that "reads" the page out loud
@media screen and (min-width: 480px) { CSS-Code; }
- translate()The translate() moves an element from its current position (according to the parameters given for the X-axis and the Y-axis).translateX()translateY()
transform: translate(5px,10px) - rotate()The rotate() method rotates an element clockwise or counter-clockwise according to a given degree.rotateX()rotateY()rotateZ()
transform: rotate(180deg) - scale()The scale() method increases or decreases the size of an element (according to the parameters given for the width and height).scaleX()scaleY()
transform: scale(1, 2); >(x, y) - skew()The skew() method skews an element along the X and Y-axis by the given angles.skewX()skewY()
transform: skew(10deg, 5deg); >(x, y) - matrix()The matrix() method combines all the 2D transform methods into one.The parameters are as follow: matrix(scaleX(), skewY(), skewX(), scaleY(), translateX(), translateY())
transform: matrix(1, -0.3, 0, 1, 0, 0);
- BasicTo create a transition effect, you must specify two things:1. the CSS property you want to add an effect to2. the duration of the effect
- transition-delayThe transition-delay property specifies a delay (in seconds) for the transition effect.
transition-delay: 1s; - transition-durationSpecifies how many seconds or milliseconds a transition effect takes to complete
transition: 2s; - transition-propertySpecifies the name of the CSS property the transition effect is for
transition: width 2s, height 2s, transform 2s; - transition-timing-functionease - specifies a transition effect with a slow start, then fast, then end slowly (this is default)linear - specifies a transition effect with the same speed from start to endease-in- specifies a transition effect with a slow startease-out- specifies a transition effect with a slow endease-in-out- specifies a transition effect with a slow start and endcubic-bezier(n,n,n,n)- lets you define your own values in a cubic-bezier function
- Basic@keyframes - Specifies the animation codeanimation-name - Specifies the name of the @keyframes animationanimation-duration - Specifies whether an animation should be played forwards, backwards or in alternate cycles
- delay & iteration-countThe transition-delay property specifies a delay (in seconds) for the transition effect.
animation-delay: 2s;animation-iteration-count: infinite; - directionnormal- The animation is played as normal (forwards). This is defaultreverse - The animation is played in reverse direction (backwards)alternate - The animation is played forwards first, then backwardsalternate-reverse - The animation is played backwards first, then forwards
- Shorthandanimation: example 5s linear 2s infinite alternate;
name duration timing-function delay iteration-count direction - fill-modenone - Default value. Animation will not apply any styles to the element before or after it is executingforwards - The element will retain the style values that is set by the last keyframe (depends on animation-direction and animation-iteration-count)backwards - The element will get the style values that is set by the first keyframe (depends on animation-direction), and retain this during the animation-delay periodboth - The animation will follow the rules for both forwards and backwards, extending the animation properties in both directions
- timing-functionease - specifies a animations effect with a slow start, then fast, then end slowly (this is default)linear - specifies a animations effect with the same speed from start to endease-in- specifies aanimationsn effect with a slow startease-out- specifies a animations effect with a slow endease-in-out- specifies a animations effect with a slow start and endcubic-bezier(n,n,n,n)- lets you define your own values in a cubic-bezier function
- Basic-webkit-mask-image: url( );-webkit-mask-repeat: no-repeat;
- Gradients-webkit-mask-image: linear-gradient(black, transparent);-webkit-mask-image: radial-gradient(circle, black 50%, rgba(0, 0, 0, 0.5) 50%);
- SVG< svg width="600" height="400">< mask id="svgmask">< polygon fill="#ffffff" points="200 0, 400 400, 0 400">< /polygon>< /mask>< image xmlns:xlink=" " xlink:href=" " mask="url(#svgmask)"> < /image>< /svg>
- Basic1. Block, for sections in a webpage2. Inline, for text3. Table, for two-dimensional table data4. Positioned, for explicit position of an element
syntaxdisplay: flex; - flex-flowflex-direction: column;flex-wrap: wrap;
shorthandflex-flow: row wrap; - alignjustify-content: center;justify-content — controls alignment of all items on the main axisalign-items: center;>align-items— controls alignment of an individual flex item on the cross axis.align-content: stretch;Modifies the behavior of the flex-wrap property.
- order1. The order property specifies the order of the flex items.2. The order value must be a number, default value is 0.
syntaxstyle="order: 3" - flex1. The flex property is a shorthand property for the flex-grow, flex-shrink, and flex-basis properties.2. The flex-grow property specifies how much a flex item will grow relative to the rest of the flex items.3. The flex-shrink property specifies how much a flex item will shrink relative to the rest of the flex items.4. The flex-basis property specifies the initial length of a flex item.
style="flex: 1 2 200px" - align-selfSpecifies the alignment for a flex item (overrides the flex container's align-items property)
syntaxstyle="align-self: flex-start"
- Basic1. An HTML element becomes a grid container when its display property is set to grid or inline-grid.2. All direct children of the grid container automatically become grid items.
syntaxdisplay: grid;display: inline-grid; - gapWe can adjust the gap size by using one of the following properties:1. row-gap : 50px2. column-gap : 50px
shorthandgap : 50px 100px; ( row column ) - Specifies itemgrid-row-start : 1grid-row-end : 3shorthand: grid-row: 1 / 3grid-column-start : 1grid-column-end : 3shorthand: grid-column: 1 / 3
shorthandgrid-area: 1 / 2 / 5 / 6;( row-start / column-start / row-end / column-end ) - grid-template-columns1. The grid-template-columns property defines the number of columns in your grid layout, and it can define the width of each column.2. The value is a space-separated-list.
syntaxgrid-template-columns: 80px 200px auto 40px; - grid-template-rows1. The grid-template-rows property defines the number of rows in your grid layout, and it can define the height of each rows.2. The value is a space-separated-list.
syntaxgrid-template-rows: 80px 200px - Good to Know1. grid-column: 1 / 5; here start on column 1 and end before column 5:2. grid-column: 1 / span 5; here start on column 1 and span 5 columns:3. grid-template-areas: 'header header header''menu main right''menu footer footer'
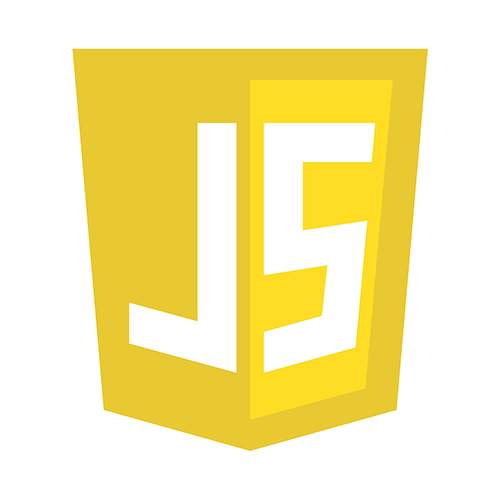
Java Script
- Keywords
Keyword Description var Declares a variable let Declares a block variable const Declares a block constant - Lengthlet text = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";let length = text.length;
- Objectsconst car = {type:"Fiat", model:"500", color:"white"};car.type()
- DOMdocoment.getElementById('navbar');
getElementsByClassName('item');
querySelector('.item' / '#item');
querySelectorAll('li'); - Output1. Writing into an HTML element, using innerHTML .document.getElementById( "id" ).innerHTML = 5 + 6;2. Writing into the HTML output using document.write() .document.write(5 + 6);3. Writing into an alert box, using window.alert() .We can skip the window keyword.4. Writing into the browser console, using console.log() .
- Events
Event Description onchange An HTML element has been changed onclick The user clicks an HTML element onmouseover The user moves the mouse over an HTML element onmouseout The user moves the mouse away from an HTML element onkeydown The user pushes a keyboard key onload The browser has finished loading the page
- varThe var keyword should only be used in code written for older browsers.Only use var if you MUST support old browsers.
syntaxvar x = 5;var y = 6;var z = x + y; - letThe let keywords were added to JavaScript in 2015.Only use let if you can't use const
syntaxlet x = 5;let y = 6;let z = x + y; - constThe const keywords were added to JavaScript in 2015.Alway use const if the value should not be changed.
syntaxconst x = 5;const y = 6;let z = x + y; - Stringlet color = "black";let Name = "Aditya";
- Numberlet length = 16;let weight = 7.5;
- Booleanlet x = true;let y = false;
- Objectconst person = {firstName:"John", lastName:"Doe"};
- Array objectconst cars = ["Saab", "Volvo", "BMW"];
- Date objectconst date = new Date("2022-03-25");
- Undefinedlet car;
- Null
- Arithmetic Operators
Operators Name Example + Addition x + y - Subtraction x - y * Multiplication x * y / Division x / y % Modulus (Remainder) x % y ++ Increment x ++ y -- Decrement x -- y - Assignment Operators
Operators Example Same As = x = 10 y = 5 += x += 5 x = x + 10 -= x -= 5 x = x - 10 *= x *= 5 x = x * 10 /= x /= 5 x = x / 10 %= x %= 5 x = x % 10 **= x **= 5 x = x ** 10 - Comparison Operators
Operators Name Example == Equal to x == y === equal value & type x === y != Not Equal x != y > Greater than x > y < Less than x < y >= Greater than or equal to x >= y <= Less than or equal to x <= y - Logical Operators
Operators Name Example && Logical and x < 5 && x < 10 || Logical or x < 5 || x < 4 ! Logical Not !(x < 5 && x < 10) - Bitwise operators
Operators inpu1 inpu2 Output & 0101 0110 0100 | 0101 0110 0111 ^ 0101 0110 0011 ~ 0101 1010 << 0101 0001 1010 >> 0101 0001 0010 - ifUse if to specify a block of code to be executed, if a specified condition is trueif (condition) {}
- elseUse else to specify a block of code to be executed, if the same condition is falseif (condition) {} else { }
- else ifUse else if to specify a new condition to test, if the first condition is falseif (condition) {} else if (condition2) {} else {}
- For Looplet i = 0;for ( i = 0; i < 5; i++) {console.log(i);}
- For in Loopconst person = {fname:"Aditya", lname:"Roy", age:24};let txt = "";for (let x in person) {console.log(txt += person[x] + " ");}
- For of Looplet language = "JavaScript";let text = "";for (let x of language) {console.log(text += x);}
- while loopslet j = 0;while (j<4) {console.log(`${j} is less then 4`);j++;}
- Do While Looplet k=6;do {console.log(`${k});k++;}while (k < 5);
- Syntaxlet day = 1;switch(day) {case 0:break;case 1:break;default:}
- Breakfor (let i = 0; i < 10; i++) {if (i == 4) {break;}console.log(text += "The number is " + i + "
");}
for loop break and jumps out when i is equal to 4: - Continuefor (let i = 0; i < 10; i++) {if (i == 4) {Continue;}console.log(text += "The number is " + i + "
");}
This example skips the value of 4: - Syntax1. const cars = ["Saab", "Volvo", "BMW"];2. let name=new Array(41,2,4,"Aditya",undefined);
Arrays are used to store multiple values or Elements in a single variable. - Access the Elementsconst cars = ["Adi", "Volvo", "BMW"];let car = cars[0];console.log(car)
Outputs Adi - Change an Array Elementconst cars = ["Adi", "Volvo", "BMW"];cars[0] = "Roy";console.log(cars)
Outputs Roy - Array Methods
Methods Methods length toString() pop() push() shift() unshift() join() delete() concat() flat() splice() slice() - Sortingconst fruits = ["Banana", "Orange", "Apple", "Mango"];fruits.sort();
reverses the elementsfruits.reverse(); - Syntaxlet name = "Aditya Roy";let text = ` hi ${name} `;
Arrays are used to store multiple values or Elements in a single variable. - escape sequences
Code Result \' ' -Single quote \" " -Double quote \\ \ -Backslash /b Backspace /f Form Feed /n New Line - String Methods
Methods Methods Methods length slice() substring() substr() replace() replaceAll() toUpperCase() toLowerCase() concat() trim() charAt() split() charCodeAt() trimStart() padStart() - String Search
Search Methods index index of the firdt indexOf() index of the last lastIndexOf() searches for a string search() To match match() string contains or not includes() start with ot not startsWith() end with ot not endsWith() - Syntaxfunction myFunction(a, b) { // Createreturn a * b;}let x = myFunction(4, 3);
- Local Variablesfunction myFunction() {let carName = "Volvo";}
- arrow functionlet sum =(a,b)=>{return a+b;}
- ways to createnewDate()newDate(date string)
newDate(year,month)newDate(year,month,day)newDate(year,month,day, hours,minutes,seconds ,ms)
newDate(milliseconds) - Get Date Method
Method Description getFullYear() four digit number (yyyy) getMonth() number (0-11) getDate() number (1-31) getDay() number (0-6) getHours() Get hour (0-23) getMinutes() Get minute (0-59) getSeconds() Get second (0-59) getMilliseconds() Get millisecond (0-999) getTime() milliseconds since January 1, 1970 - Set Date Methods
Method Description setFullYear() year (optionally month and day) setMonth() number (0-11) setDate() number (1-31) setDay() number (0-6) setHours() Get hour (0-23) setMinutes() Get minute (0-59) setSeconds() Get second (0-59) setMilliseconds() Get millisecond (0-999) setTime() milliseconds since January 1, 1970 - Properties (Constants)
Math.property The syntax for any Math property Math.E returns Euler's number Math.PI returns PI Math.SQRT2 returns the square root of 2 Math.SQRT1_2 returns the square root of 1/2 Math.LN10 returns the natural logarithm of 10 Math.LOG2E returns base 2 logarithm of E - Math Methods
Math.method(x) The syntax for Math any methods Math.round(x) Returns x rounded to its nearest integer Math.ceil(x) Returns x rounded up to its nearest integer Math.floor(x) Returns x rounded down to its nearest integer Math.pow(x, y) returns the value of x to the power of y: Math.sqrt(x) returns the square root of x: Math.abs(x) returns the absolute (positive) value of x: Math.sin(x) returns the sine of the angle x Math.cos(x) returns the cosine of the angle x Math.min() can be used to find the lowest value in a list Math.max() can be used to find the highest value in a list Math.random() returns a random number between 0 and 1 - Syntaxlet jsonobj={ //objname :"aditya",Aka: "Atomic",food: "meat"}
- obj to stingAs Syntax
let myjosnstr=JSON.stringify (jsonobj); - replace stringAs Syntax
let myjosnstr=JSON.stringify(jsonobj);myjosnstr =myjosnstr.replace ('meat','fish') - string to objAs Syntax
let myjosnstr=JSON.stringify (jsonobj);myjosnstr =myjosnstr.replace ('meat','fish')newjsonobj=JSON.parse (myjosnstr)